How to Upload an Android Studio Project to a Gitub Repository

Very few Android projects are an island! The majority of Android projects have dependencies on a number of other components, including third party Android libraries.
An Android library contains the same files you'd find in a regular Android project, such as source code, resources, and a Manifest. Even so, instead of compiling into an Android Package Kit (APK) that tin can run on an Android device, a library compiles into a code annal that you can use equally a project dependency. These libraries give you access to a wide range of additional functionality, including some features that aren't included in the vanilla Android platform.
Ane of the best places to find Android libraries, is GitHub. However, getting a library from its GitHub page and into your project isn't ever straightforward, especially since there's several different repositories that developers can utilize to distribute their GitHub projects – and it may not ever be obvious which repository a programmer is using!
In this article, I'one thousand going to show you how to import whatever library you lot detect on GitHub into your Android project, regardless of whether you desire to add the library every bit a remote dependency, or as a local dependency.
Adding remote dependencies
Android Studio's Gradle build arrangement adds libraries to your projection as module dependencies. These dependencies can either be located in a remote repository, such equally Maven or JCenter, or they can be stored within your projection, equally a local dependency – you lot only need to let Gradle know where it can notice these dependencies.
Adding a library as a remote dependency is typically the quickest and easiest way of getting a library's code into your project, and then this is the method we're going to look at first. When you lot add a library as a remote dependency, Gradle will make certain that dependency has everything it needs to able to run, including any transitive dependencies, and so yous'll typically want to add a library as a remote dependency wherever possible.
To add together a remote dependency, you'll need to provide Gradle with two pieces of information:
- The repository. Gradle needs to know the repository (or repositories) where it should look for your library (or libraries). Android libraries tend to exist distributed via either Maven Central or JCenter.
- The compile statement. This contains the library's bundle name, the name of the library's group, and the version of the library you want to apply.
Ideally, the library's GitHub page should provide yous with all this data. In reality this isn't always the case, but let'due south beginning with the all-time instance scenario and assume that the library's GitHub folio does include this data.
Adding a remote dependency with JCenter
StyleableToast is a library that lets you customize every part of Android's toasts, including changing the background colour, corner radius and font, and adding icons. It also provides all the information you lot need to add this library to your project, in its dedicated 'Installation' section. Hither, we can see that this projection is distributed via JCenter.
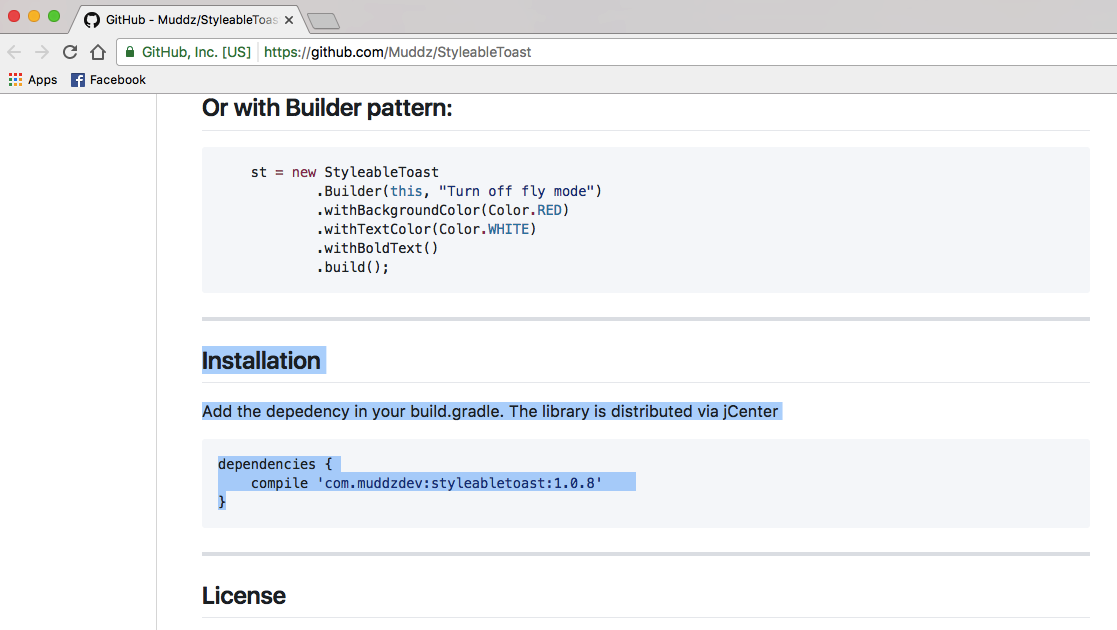
When you create a project with the latest releases of Android Studio, your projection'southward build.gradle files are already setup to support JCenter. If yous open your project-level build.gradle file, then you'll meet that JCenter is already included in the 'allprojects / repositories' section:
Code
allprojects { repositories { jcenter() } }
Note, the project-level build.gradle file contains two 'repositories' blocks, merely the 'buildscript / repositories' block is where you define how Gradle performs this build. You lot shouldn't add any module dependencies to this department.
Since your projection is already configured to check JCenter, the merely thing we need to practise is add our compile statement to the module-level build.gradle file.
Once again, StyleableToast provides the states with exactly the data we demand, then just copy the compile statement from StyleableToast's GitHub page, and paste information technology into your Gradle file:
Code
dependencies { compile 'com.muddzdev:styleabletoast:1.0.8' }
Sync your Gradle files, either by clicking the 'Sync' banner, or by selecting the 'Sync Project with Gradle Files' icon in the toolbar. Gradle will then query the JCenter server to cheque that the Styleabletoast library exists, and download all of its files. You're at present set up to start using this library!
2. Calculation a remote dependency with Maven Cardinal
Alternatively, if the project'south GitHub page states that this library is distributed via Maven Fundamental, and so you lot'll demand to tell Gradle to check Maven Cardinal instead.
Open up your project-level build.gradle file and add Maven Central to the "allprojects" block:
Code
allprojects { repositories { mavenCentral() } }
From here, the rest of the process is exactly the same: open up your module-level build.gradle file, add the compile statement and sync with Gradle.
3. Adding a remote dependency that's hosted on its own server
Occasionally, you may encounter a projection that's still distributed via JCenter or Maven Fundamental, but the developer has chosen to host their project on their own server. When this is the case, the project's GitHub page should tell you to utilise a very specific URL, for case Textile's Crashlytics Kit repository is located at https://maven.fabric.io/public.
If you see this kind of URL, and so you'll need to open your project-level build.gradle file, and and so declare the repository (in this instance, Maven) along with the exact URL:
Code
repositories { maven { url 'https://maven.cloth.io/public' } }
You tin then add the compile statement and sync your files equally normal.
What if I can't find the repository and/or compile statement?
Upwardly until now, we've been optimistic and causeless that the project'southward GitHub e'er tells yous all the data you lot need to know. Unfortunately this isn't always the case, so let'south shift from the all-time instance scenario, to the worst case scenario, and imagine that the project'south GitHub page doesn't provide y'all with any information about the repository and compile argument you need to use.
In this scenario, you can either:
- Apply JitPack.
- Clone the entire repository, and import its code into your projection equally its own module.
Using JitPack
JitPack is a package repository for Git that lets you add together any GitHub project as a remote dependency. As long as the library contains a build file, JitPack can generate all the data you need to add together this library to your project.
The first step, is to open your project-level build.gradle file and add together JitPack every bit a repository:
Code
allprojects { repositories { maven { url 'https://jitpack.io' } } }
You can and then use the JitPack website to generate a compile argument, based on that project's GitHub URL:
- In your web browser, navigate to the library's GitHub folio. Copy its URL.
- Head over to the JitPack website.
- Paste the URL into the website'due south search field and then click the accompanying 'Wait Up' push button.
- The webpage volition then brandish a table of all the versions of this library, split across diverse tabs: Releases, Builds, Branches and Commits. Typically, Releases tend to be more than stable, whereas the Commit department contains the latest changes.
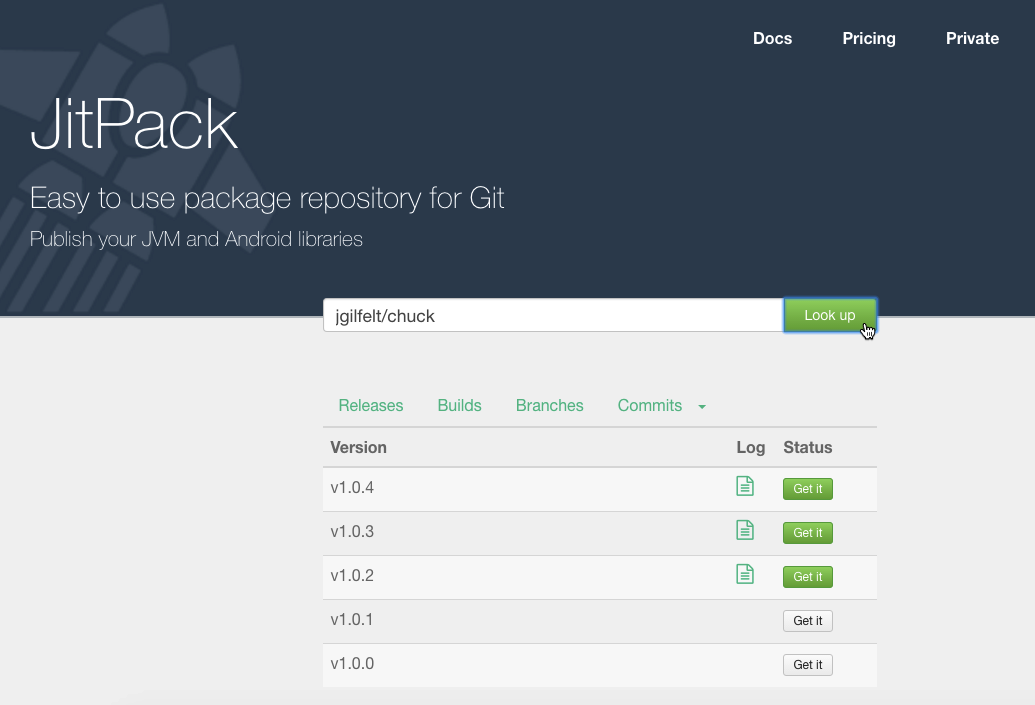
- One time you've decided what version you want to use, click its accompanying 'Get Information technology' button.
- The website should update to display the exact compile statement you lot need to use.
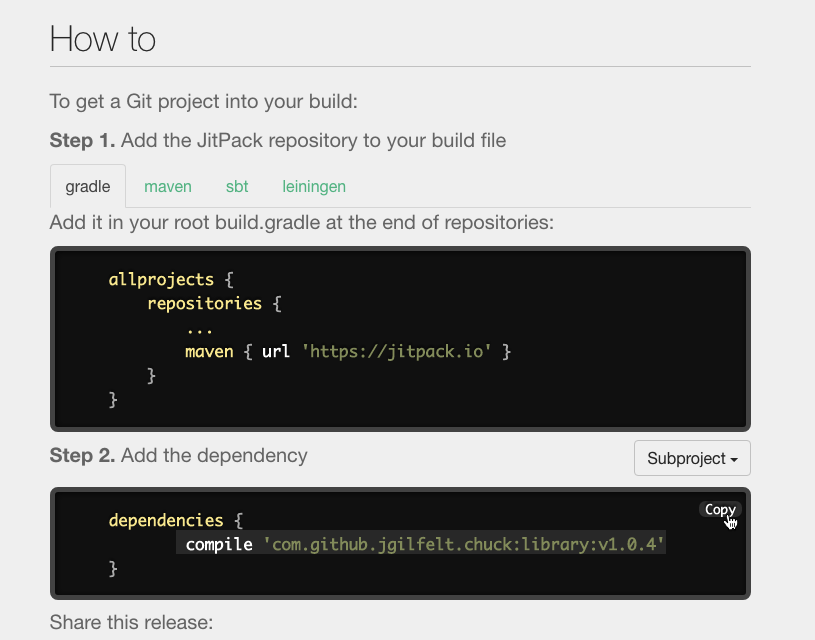
- Copy/paste this compile statement into your project'due south module-level build.gradle file.
- Sync your Gradle files, and you're ready to first using your library!
Cloning a GitHub project
Alternatively, when you're unsure of a library's repository and/or compile argument, you lot may desire to clone the GitHub project. Cloning creates a re-create of all the GitHub project's code and resources, and stores this copy on your local machine. You tin then import the clone into your project as its own module, and utilize it equally a module dependency.
This method tin can be time-consuming, and importing the entirety of a projection'south lawmaking may cause conflicts with the residuum of your projection. However, cloning does requite you lot admission to all of the library'southward code, so this method is ideal if you want to customize the library, for instance by tweaking its code to better integrate with the rest of your project, or fifty-fifty adding new features (although if y'all feel like other people might benefit from your changes, then you may want to consider contributing your improvements back to the project).
To clone a GitHub projection:
- Create a GitHub business relationship.
- Select 'Checkout from Version Control' from Android Studio'southward 'Welcome' screen.
- Enter your GitHub credentials.
- Open your spider web browser, navigate to the GitHub repository you desire to clone, and and then re-create/paste its URL into the Android Studio dialog.
- Specify the local directory where yous want to shop the cloned repository.
- Requite this directory a name, and then click 'Clone.'
Now you lot accept a re-create of the library's code, you tin can import this library into your Android project, as a new module:
- Open the project where yous want to apply your cloned library, and then select 'File > New > Import Module' from the Android Studio toolbar.
- Click the three-dotted button and navigate to your cloned repository. Select this repository, so click 'OK.'
- Click 'Terminate.'
- Select 'File > Project structure' from the Android Studio toolbar.
- In the left-hand carte, select the module where y'all desire to use this library.
- Select the 'Dependencies' tab.
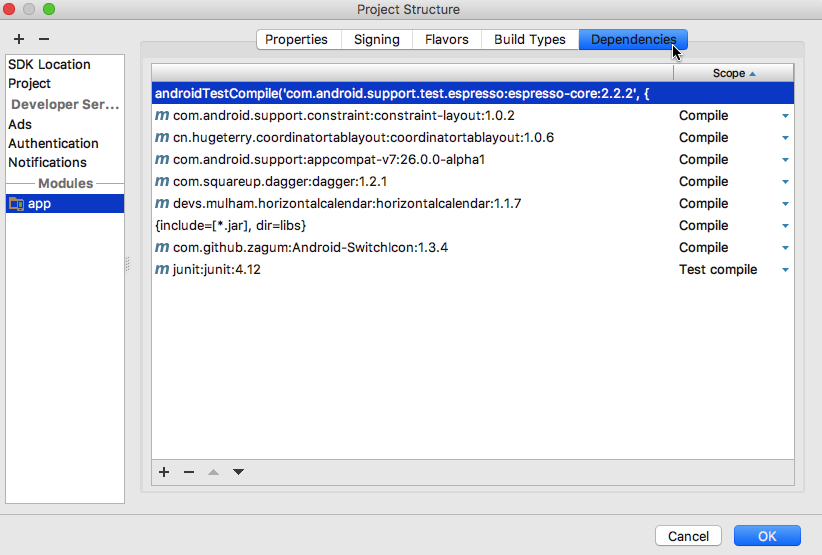
- Select the little '+' icon, followed by 'Module dependency.'
- Select your library module, then click 'OK.'
- Go out the 'Project construction' window.
Depending on the library yous're using, you may need to make some adjustments to your imported code earlier your project will compile. For example if Android Studio's 'Messages' tab is complaining about incompatible minSdkVersions, then chances are the APIs beingness used past the library aren't compatible with the versions of the Android platform defined in your project'southward build.gradle file. Similarly, if Android Studio is complaining about your projection's buildToolsVersion, then it's likely there'south a mismatch between the version defined in the library and the version defined elsewhere in your projection. In both of these scenarios, you'll need to cheque the values defined in both build.gradle files, and change them accordingly.
Troubleshooting
When you're working with any kind of third party software, equally a general rule you lot're more likely to encounter incompatibilities, bugs, and all-around strange beliefs, compared to when you lot're using a suite of software that was adult by the same squad, and where every slice of the puzzle was specifically designed to work together.
If y'all practice run into bug after adding a library to your projection, then try the following fixes:
- Check that you haven't accidentally added multiple versions of the same library. If Android Studio is reporting a "multiple DEX files ascertain…" error, then you may have added the same library to your project more than once. Yous can review your module's dependencies past selecting 'File > Project structure' from the Android Studio toolbar, then selecting the module yous want to examine, and clicking the 'Dependencies' tab. If a library appears in this window multiple times, then select the indistinguishable, and click the footling '-' icon to remove information technology.
- Search the web. There'southward e'er a chance that other people may accept encountered the same upshot as you, so perform a quick Google search to run across whether anyone has posted about this issue on forums, or communities like Stackoverflow. You may even become lucky and discover a weblog or a tutorial that includes instructions on how to resolve this exact event.
- Make clean and rebuild your project. Sometimes, selecting 'Build > Make clean project' from the Android Studio toolbar, followed by 'Build > Rebuild project,' may be plenty to solve your problem.
- And if all else fails… Getting third party software to work correctly sometimes requires a fleck of trial and fault, so if there's an alternate method of importing your called library, then it'south always worth trying. But because your project is refusing to compile after you lot've imported a cloned repository, doesn't necessarily mean that it's going to take the same reaction if you lot endeavor to use that same library as a remote dependency.
Wrapping Upwardly
In this commodity, nosotros looked at how you can add any library y'all notice on GitHub, to your Android project, regardless of whether that library is distributed via JCenter or Maven Fundamental. And, even if y'all have no idea what repository or compile statement you need to use, and then you always have the option of using JitPack, or cloning the library'south code.
Have you discovered any groovy Android libraries on GitHub? Let us know in the comments below!
Source: https://www.androidauthority.com/add-a-github-library-to-android-studio-using-maven-jcenter-jitpack-777050/
0 Response to "How to Upload an Android Studio Project to a Gitub Repository"
Post a Comment